Arduino and Processing
Duration six weeks
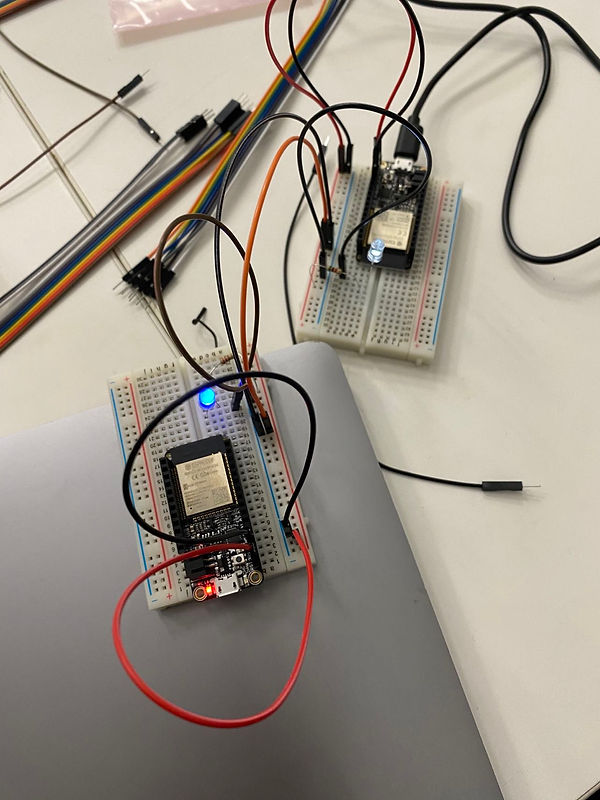
#define LED 13
#define Button 12
void setup() {
pinMode(LED,OUTPUT);
pinMode(Button,INPUT);
}
void loop() {
int ButtonState = digitalRead(Button);// read the botton pin and store in ButtonState variable
if(ButtonState == HIGH)
{
digitalWrite(LED, HIGH);
}
else
{//otherwise
digitalWrite(LED, LOW);
}
}
#define TrigPin 7
#define EchoPin 8
float Value_cm;
void setup(){
Serial.begin(9600);
pinMode(TrigPin, OUTPUT);
pinMode(EchoPin, INPUT);
}
void loop(){
digitalWrite(TrigPin,LOW);
delayMicroseconds(2);
digitalWrite(TrigPin,HIGH);
delayMicroseconds(10);
digitalWrite(TrigPin,LOW);
Value_cm = float(pulseIn(EchoPin,1)*17)/1000;
Serial.println(Value_cm);
//Serial.println("cm");
//int Val = Value_cm;
//Serial.write(Val);
delay(100);
}
Arduino code
Set up the distance sensor, and get data as distance as 'cm'
and send the data to processing
Processing code
Using data from Arduino as variables in the drawing function, and transfers to different color hues.
import processing.serial.*;
Serial myPort;
float val;
float colorBG;
void setup()
{
size(300,300);
String portName = Serial.list()[1];
myPort = new Serial(this, portName, 9600);
myPort.bufferUntil('\n');
}
void draw()
{
//if(myPort.available() >0){
// val = myPort.read();
//}
colorBG = val*2;
colorMode(HSB, random(300,354), 300 , random(1.300));
background(colorBG, 2*colorBG, colorBG*3 );
text(val, height/2, width/2);
fill(colorBG, colorBG*2);
rect(50, 50, 100, 100);
}
void serialEvent(Serial p){
val = float(p.readString());
}
Arduino processing instrument
Using Arduino and processing to make a piano. Use a capacitive sensor as the key of the piano. When humans touch the wire, the electronic signal will automatically transfer to the Arduino
#include <CapacitiveSensor.h>
​
CapacitiveSensor cs_2_3 = CapacitiveSensor(2,3);
CapacitiveSensor cs_2_4 = CapacitiveSensor(2,4);
CapacitiveSensor cs_2_5 = CapacitiveSensor(2,5);
CapacitiveSensor cs_2_6 = CapacitiveSensor(2,6);
CapacitiveSensor cs_2_7 = CapacitiveSensor(2,7);
CapacitiveSensor cs_2_8 = CapacitiveSensor(2,8);
CapacitiveSensor cs_2_9 = CapacitiveSensor(2,9);
CapacitiveSensor cs_2_10 = CapacitiveSensor(2,10);
void setup()
{
cs_2_3.set_CS_AutocaL_Millis(0xFFFFFFFF);
Serial.begin(115200);
Serial.println("ready");
}
void loop()
{
// Set a timer.
long start = millis();
// Set the sensitivity of the sensors.
long total1 = cs_2_3.capacitiveSensor(3000);
long total2 = cs_2_4.capacitiveSensor(3000);
long total3 = cs_2_5.capacitiveSensor(3000);
long total4 = cs_2_6.capacitiveSensor(3000);
long total5 = cs_2_7.capacitiveSensor(3000);
long total6 = cs_2_8.capacitiveSensor(3000);
long total7 = cs_2_9.capacitiveSensor(3000);
long total8 = cs_2_10.capacitiveSensor(3000);
//
// Serial.println(millis() - start);
// Serial.print(',');
Serial.print(total1);
Serial.print(",");
Serial.print(total2);
Serial.print(",");
Serial.print(total3);
Serial.print(",");
Serial.print(total4);
Serial.print(",");
Serial.print(total5);
Serial.print(",");
Serial.print(total6);
Serial.print(",");
Serial.print(total7);
Serial.print(",");
Serial.println(total8);
​
delay(10);
}
Arduino code
Set up Capacitive Sensor inside Arduino, and get each sensor's data.
As the piano has eight keys, so get eight different data.

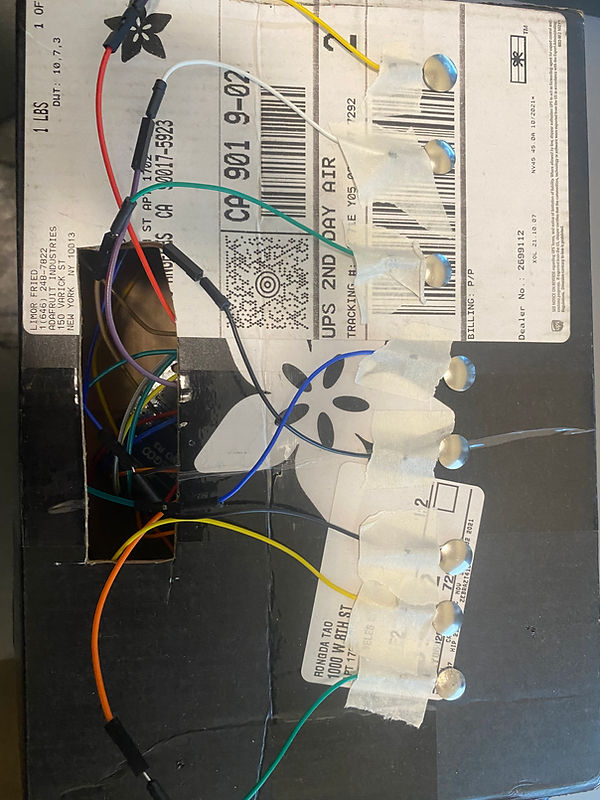
import processing.serial.*;
import processing.sound.*;
SoundFile Do;
SoundFile Re;
SoundFile Mi;
SoundFile Fa;
SoundFile Sol;
SoundFile La;
SoundFile Si;
SoundFile Do1;
Serial mySerial;
//String portName = Serial.list()[1];
float keyone = 0.0;
float keytwo = 0.0;
float keythree = 0.0;
float keyfour = 0.0;
float keyfive = 0.0;
float keysix = 0.0;
float keyseven = 0.0;
float keyeight = 0.0;
////int nl = 10;
int isPlay = 0;
int isPlay1 = 0;
int isPlay2 = 0;
int isPlay3 = 0;
int isPlay4 = 0;
int isPlay5 = 0;
int isPlay6 = 0;
int isPlay7 = 0;
void setup() {
size(800, 800);
colorMode(HSB);
Do = new SoundFile(this, "do.wav");
Re = new SoundFile(this, "re.wav");
Mi = new SoundFile(this, "mi.wav");
Fa = new SoundFile(this, "fa.wav");
Sol = new SoundFile(this, "sol.wav");
La = new SoundFile(this, "la.wav");
Si = new SoundFile(this, "si.mp3");
Do1 = new SoundFile(this, "do1.wav");
mySerial = new Serial(this, Serial.list()[1], 115200);
mySerial.bufferUntil('\n');
}
​
void draw() {
background(0);
fill(keytwo, 255, 255);
circle(width/2, height/2, keyone);
​
if (keyone >= 2000 && isPlay == 0) {
//background(0);
Do.play();
isPlay = 1;
}
else if (keyone < 2000) {
//snareDrum.stop();
isPlay = 0;
}
if(keytwo >= 2000 && isPlay1 == 0) {
//background(0);
Re.play();
isPlay1 = 1;
} else if(keytwo < 2000){
//snareDrum.stop();
isPlay1 = 0;
}
if(keythree >= 2000 && isPlay2 == 0) {
//background(0);
Mi.play();
isPlay2 = 1;
} else if(keythree < 2000){
//snareDrum.stop();
isPlay2 = 0;
}
if(keyfour >= 2000 && isPlay3 == 0) {
//background(0);
Fa.play();
isPlay3 = 1;
} else if(keyfour < 2000){
//snareDrum.stop();
isPlay3 = 0;
}
if(keyfive >= 2000 && isPlay4 == 0) {
//background(0);
Sol.play();
isPlay4 = 1;
} else if(keyfive < 2000){
//snareDrum.stop();
isPlay4 = 0;
}
if(keysix >= 2000 && isPlay5 == 0) {
//background(0);
La.play();
isPlay5 = 1;
} else if(keysix < 2000){
//snareDrum.stop();
isPlay5 = 0;
}
if(keyseven >= 2000 && isPlay6 == 0) {
//background(0);
Si.play();
isPlay6 = 1;
} else if(keyseven < 2000){
//snareDrum.stop();
isPlay6 = 0;
}
if(keyeight >= 4000 && isPlay7 == 0) {
//background(0);
Do1.play();
isPlay7 = 1;
} else if(keyeight < 4000){
//snareDrum.stop();
isPlay7 = 0;
}
Processing code
Import Data from Arduino, then Import sound file in the data file, define the variables.
Define when to play the sound, and when to stop the sound.
void serialEvent(Serial p) {
String transmission = p.readString();
String cleanedUpTransmission = trim(transmission);
float[] values = float(split(cleanedUpTransmission, ','));
if (values.length == 8) {
keyone = values[0];
keytwo = values[1]; //, 0, 1000000, 0, 5000);
keythree = values[2];
keyfour = values[3];
keyfive = values[4];
keysix = values[5];
keyseven = values[6];
keyeight = values[7];
}
//println(values);
}